There are two existing ways to format Strings. One is string modulo operator(%) and the other is str.format(value). The former is old and the latter is relatively new.
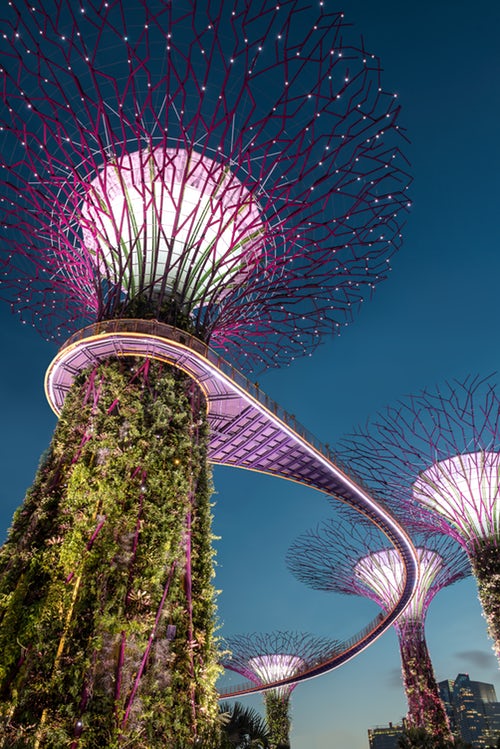
- Syntax: {}.format(value)
- (value) : Can be an integer, floating point numeric constant, string, characters or even variables.
1. string modulo operator(%)
#2
format = "there is %s culture among %s"
wecode = ("implict-rule", "wecoders")
print(format % wecode)
2. str.format(value)
#1
wecode = 'We are {} who believe are creating wealth every time we write {}'.format("wecoders", "code")
print(wecode)
#2
wecode = '{0} are writing {1} '.format("wecoders", "code")
print(wecode)
There are so many cool stuff coming out every day in programming languages and Python is no different. Why don’t you try more new, easy and improved way to format Strings in Python.
1. Formatted string literals
Formatted string literals was joined in Python 3.6, which was written by Eric V. Smith in August of 2015. F-strings are string literals which have an f at the beginning and curly braces containing expressions that will be replaced with their values. f-strings are known to be faster than old versions of both %-formatting and str.format()
name = "Jason Mraz"
age = 32
#LowerCase
print(f"Hello, I'm {name} and I'm {age} years old")
#UpperCase
print(F"Hello, I'm {name} and I'm {age} years old")
You can also interchangeably use capital letter F. It’s pretty simple and familiar to someone who’s pro at Javascript. ${variable}
Plus, there are multiple use cases of f-strings.
- Method with strings.
name = "jason mraz"
job = "SINGER-SONGWRITER"
print(f"{name.upper()} is a great {job.lower()}!!")
- Call functions
def to_lowercase(input):
return input.lower()
print(to_lowercase('JASON MRAZ'))
- Multiline f-strings and line break
- You always need to put an f(or F) in front of each line of a multiline string in order for Python to read code properly.
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
profile = (f"He is {name}",
F"a {job}, who first came to prominence in San Diego in 2000 and"
F"his debut album was {debut_album}"
)
print(profile)
#('He is Jason Mraz', 'a singer-songwriter, who first came to prominence in San Diego in 2000 andhis debut album was Jason Mraz Live & Acoustic 2001')
What is there is no parentheses in the start and end sentences?? Python only reads the first line of a sentence and ignore the following line of sentences.
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
profile = f"He is {name}",
F"a {job}, who first came to prominence in San Diego in 2000 and"
F"his debut album was {debut_album}"
print(profile)
#('He is Jason Mraz',)
To make the code interpreted, you need to use backslash \ in the end of every sentence so that you can spread strings over multiple lines.
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
profile = f"He is {name}, "\
F"a {job}, who first came to prominence in San Diego in 2000 and " \
F"his debut album was {debut_album}"\
print(profile)
# He is Jason Mraz, a singer-songwriter, who first came to prominence in San Diego in 2000 and his debut album was Jason Mraz Live & Acoustic 2001
But, the better way is to use three double quote """ and use just one f in the first sentence.
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
profile = f"""
He is {name}, a {job},
who first came to prominence
in San Diego in 2000 and his debut album was {debut_album}
"""
print(profile)
#He is Jason Mraz, a singer-songwriter,
#who first came to prominence
#in San Diego in 2000 and his debut album was Jason Mraz Live & Acoustic 2001
Dictionaries
Be careful with quotation marks when using f-strings. If you want to use a single quotation marks for the keys of the dictionary, you should use different quotation marks. f-strings for a single quotations marks and calling for dictionaries for double quotations marks. Or vice versa.
#different quotations marks
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
Jason_Mraz = {'name': "Jason Mraz", 'job': "singer-songwriter"}
print(f'His name is {Jason_Mraz["name"]} and {Jason_Mraz["job"]}')
#different quotations marks
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
Jason_Mraz = {'name': "Jason Mraz", 'job': "singer-songwriter"}
print(f"His name is {Jason_Mraz['name']} and {Jason_Mraz['job']}")
# You cannot use escape \ inside the value of dictionary in f-strings.
name = "Jason Mraz"
job = "singer-songwriter"
debut_album = "Jason Mraz Live & Acoustic 2001"
Jason_Mraz = {'name': "Jason Mraz", 'job': "singer-songwriter"}
#print(f"His name is {Jason_Mraz["\name"\]} and {Jason_Mraz["\job"\]}")