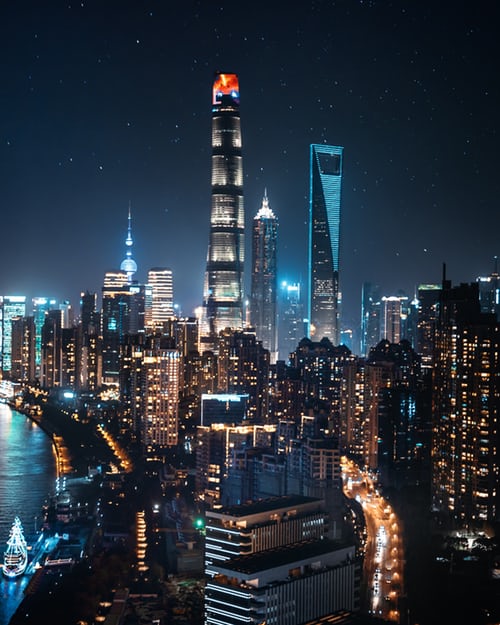
Let’s use Node.js to interact with cloud resources. Make sure node is installed on your local computer.
$ node --version
v12.13.0
Install Node.js-based Google Cloud Client Library. Enter the following command
$ sudo yarn add @google-cloud/compute
//if you are a npm user
$ sudo npm install @google-cloud/compute
Initiate Instance script.js
const GCE = require('@google-cloud/compute')
const my_instance = new GCE({projectId: 'YOUR_PROJECT_ID'})
const zone = gce.zone('asia-east1-b');
console.log('Getting your VMs...');
zone.getVMs().then((data) => {
data[0].forEach((vm) => {
console.log('Found a VM called', vm.name);
});
console.log('Done. ');
});
$ node script.js
Getting your VMs...
Found a VM called learning-cloud-demo
Done.
Stop Instance script.js
const GCE = require('@google-cloud/compute')
const my_instance = new GCE({projectId: 'sincere-loader-258805'})
const zone = my_instance.zone('asia-east1-b');
console.log('Getting your VMs...');
zone.getVMs().then((data) => {
data[0].forEach((vm) => {
console.log('Found a VM called', vm.name);
console.log('Stooping', vm.name, '...');
vm.stop((err, operation) => {
operation.on('complete', (err) => {
console.log('Stopped', vm.name);
});
})
});
});
$ node script.js
Getting your VMs...
Found a VM called learning-cloud-demo
Stooping learning-cloud-demo ...
Stopped learning-cloud-demo
The command node script.js
shows taht VM is now being stopped and can be restarted later if necessary.
We are creating wealth every time we write a code